Ok, so this semester I'm taking an advanced programming class. Basically data structures and algorithms. We've done all previous programming in C, Verilog, and other assembly languages, but now we're making the jump to Java. Our first assignment is implementing a game called Nim (look it up on Wikipedia). I already wrote sufficient code to run the entire program (albeit it was as close to C as I could possibly get), but we can earn extra points for extra features, such as:
1. error check - already implemented!
2. use classes/objects for each part (instead of programming it like C) - I haven't really messed with this too much yet
3. make graphical representation of the heaps if using the console as output - skipping this due to part 5
4. ability to pay against a computer opponent - I know how, but haven't really done anything with it yet
5. make a GUI in which to play the game - THIS IS WHAT I WANT TO DO!
So....focusing on part 5:
I have read numerous documents/tutorials on creating/using GUI's. The major problems I am having is with LayoutManagers, Buttons, Panes vs Containers, and Actions. I'll attach the code I have so far below. I get how to create new labels and such, but the Layout is really messed up. I currently am using a setLayout(null) so that I can manually place everything. This isn't a huge deal since the window is set set as not resizable. Basically I would like a window that looks like the one below. You can kind of see where I'm at by comparing my code (should compile and everything, if not, I'm using Eclipse SDK v3.5.0) to my image. I do not have the initialization in there yet though. Basically I will have a series of showInputDialog messages that will ask how many heaps they want to start with and then ask the max number of stones in a heap. Probably will just have it randomly assign a number of stones in each heap (up to the max).
So...after all that explanation and rambling...I would like some advice/samples on how to make the buttons "do stuff", and advice on how to layout the window (whether I should use a Layout Manager and if so, which one OR just place everything explicitly). Any and all help is appreciated!
Thanks,
UOMaddog
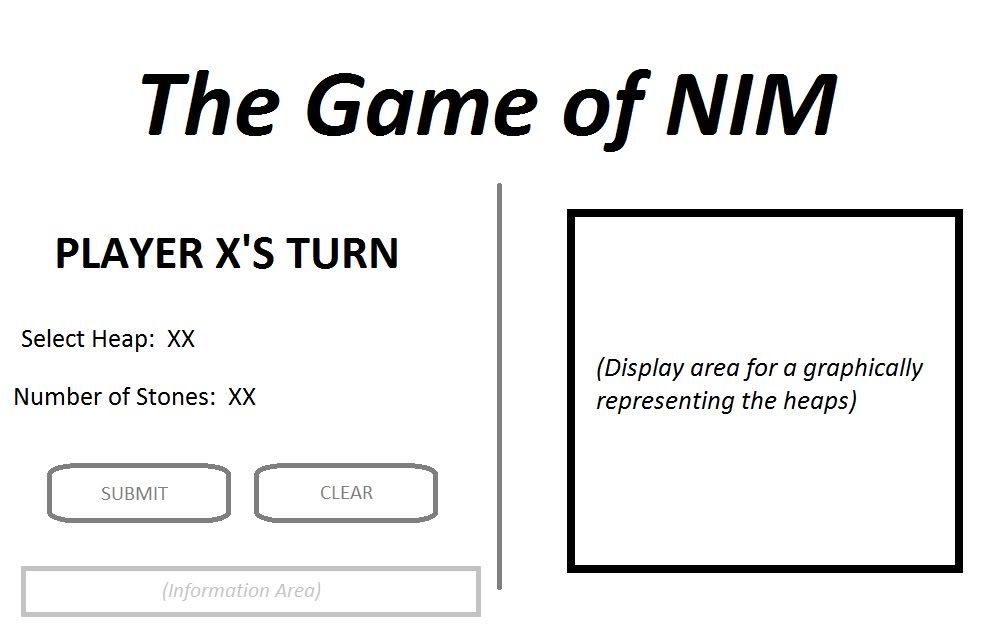
package guitest;
import java.io.*;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
import java.text.*;
import java.util.*;
public class GUItest extends JFrame {
private JLabel title;
private static JLabel currentplayer;
private static int player;
//CONSTRUCTOR FOR GUItest
GUItest() {
setTitle("NIM");
setLocation(100,100);
setSize(400,200);
setResizable(false);
setDefaultCloseOperation(EXIT_ON_CLOSE);
this.getContentPane().setLayout(null);
//TITLE
title = new JLabel("The Game of NIM");
title.setLocation(150,5);
title.setSize(95,15);
this.getContentPane().add(title);
//DISPLAY CURRENT PLAYER
player = 1;
currentplayer = new JLabel("Current Player: "+player,JLabel.LEFT);
currentplayer.setLocation(10,25);
currentplayer.setSize(100,15);
this.getContentPane().add(currentplayer);
}
//Main loop
public static void main(String args[]) throws InterruptedException {
//Generate a new window
JOptionPane.showMessageDialog(null,"NIM\nCreated by: Dominic Russo","Welcome!",JOptionPane.PLAIN_MESSAGE);
new GUItest().setVisible(true);
Thread.sleep(1000);
GUItest.player = 2;
GUItest.currentplayer.setText("Current Player: "+player);
}
}