I will do my best to help explain how namespaces interact with one another. But first, I think a picture will tell alot about how things work.
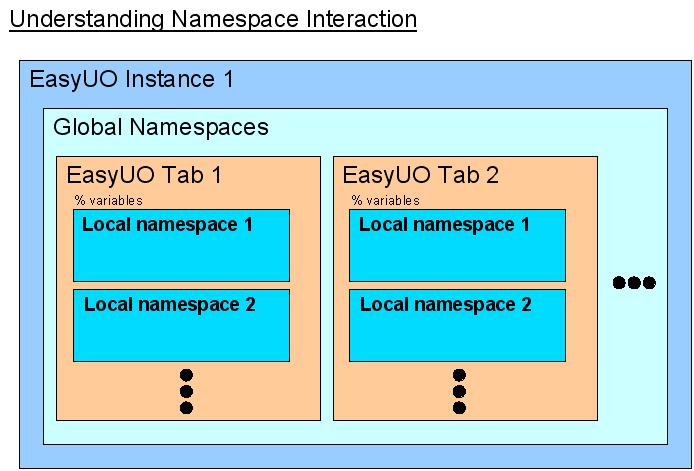
First thing you can notice is that if you have more than one instance of EUO running, the only way you can transfer information is through system variables, or "*".
But from there, it become more clear how you can transfer information from one "tab" or script to another.
Global namespaces are "global" to all the tabs running in a specific EUO instance. So you can copy information from local to global of Tab 1 and then global to local of Tab 2. I've used this method to allow scripts to interact with one another.
Finally, here's some additional information!
Why do you need namespaces?
First, namespaces are very handy to help the programmer maintain a local variable pool which can be used without fear of corrupting other variables found within their program. Mainly this is evident by subroutines working within their own namespace.
For example, here's a script with a subroutine that computes exponential powers:
set !result 2
set !count 0
while !count < 4
{
gosub POWER !result 2 ; compute !result^2
set !result #RESULT
set !count !count + 1
}
display ok !result
stop
; %1 = base, %2 = exponent
sub POWER
namespace push
namespace local POW
set !result 1
set !count 0
repeat
if %2 = 0
set !result 1
else
set !result !result * %1
set !count !count + 1
until !count >= %2
set #RESULT !result
namespace clear
namespace pop
return #RESULT
You'll see that !result and !count are used in both the function and the script, but since a new copy is used in the fuction due to the new namespace, the value contained within LOCAL:STD remains untouched. So:
gosub POWER 2 2 ; = 4
gosub POWER 4 2 ; = 16
gosub POWER 16 2 ; = 256
gosub POWER 256 2 ; = 65536
You also notice that we call "namespace clear". That's because since we won't be reusing any of the values computed in this function, we can clear all the contents. If you want to keep values computed in this function, simply omit the "namespace clear" and they will persist until the next time you call POWER.
Caution:
Be careful not to call "namespace clear" after "namespace pop". If you do, you will bring forward the last known namespace, then clear it with potential loss of data from the calling function/namespace. I have see this happen to other people before, and the results can be hard to debug.
Here's another example where a script can copy a value from one namespace to another:
set !x 5 ; start out in LOCAL:STD (scope:namespacename)
set !y 20
pause
namespace push ; remember LOCAL:STD
namespace local NS1 ; create a new namespace LOCAL:NS1
set !x 10 ; now LOCAL:NS1 has it's own !x var
namespace copy y from LOCAL STD ; need to use !y from LOCAL:NS1, now a copy is in current namespace
set !temp !x * !y
set #RESULT !temp ; store result such that it can be returned
namespace clear ; clears current namespace or LOCAL:NS1
namespace pop ; bring back last 'pushed' namespace or LOCAL:STD
set !temp !x * !y + #RESULT
display ok The result is , #SPC , !temp
stop
The answer for this is 300. You'll notice that we copy the value of !y from LOCAL:STD to complete the computation. You can also copy information *TO* a known namespace:
namespace copy y to LOCAL STD ; copy !y to LOCAL:STD
It's important to note here that you don't need the leading "!" for the copy commands since it's implied with this function.
Finally, you can do wildcard copies to and from namespaces if you need to copy more than one variable:
Copying everthing from current namespace to LOCAL:STD:
namespace copy * to LOCAL STD
Copying everthing from GLOBAL:NS1 to current namespace:
namespace copy * from GLOBAL NS1
If you only want to copy a range of items, you can build a selection based on the name:
This copies everything variable staring with "TM_" from GLOBAL:NS1 to the current namespace:
namespace copy TM_* from GLOBAL NS1
TM